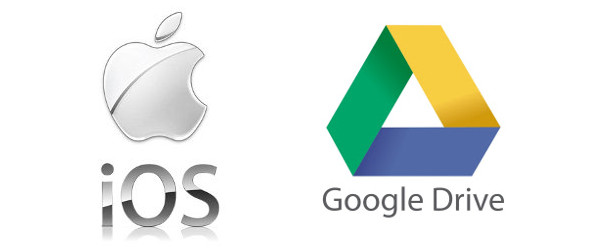
Recently I am planning to integrate the Google Drive function into my app. Meanwhile, I have to include the Google API library into my project, however I feel the documentation and the tutorial I found was not perfect enough and also, I find out another clean method to integrate the API Library together with my current project.
It is quite easy while you search the keyword “Google Drive API iOS” by google, it will highly recommend you to go to this https://developers.google.com/drive/ios/quickstart
Well, you might notice it is quite tedious doing the following steps:
Step 1: Enable Drive API
Just follow the instructions to register and what we’ll need it later is Client ID and Client Secret.
Step 2: Download Google API
It is a must to download via SVN, as the data via manual download from github is not completed.
Step 3: Create and configure an XCode project
This is the WORST part I feel it is very tricky and the documentation here is not very clear enough. Mostly people will have problem right here! Another thing I want to complain that the tutorial video is really WASTING YOUR TIME as the result come out was failed, and the speaker just opened another project to show you the result.
So I will suggest another solution at this part, which I feel clean and you don’t have to worry where to put your google-api folder and files.
From step 2, running the svn command:
svn checkout http://google-api-objectivec-client.googlecode.com/svn/trunk/ google-api-objectivec-client-read-only
you will have the folder google-api-objectivec-client-read-only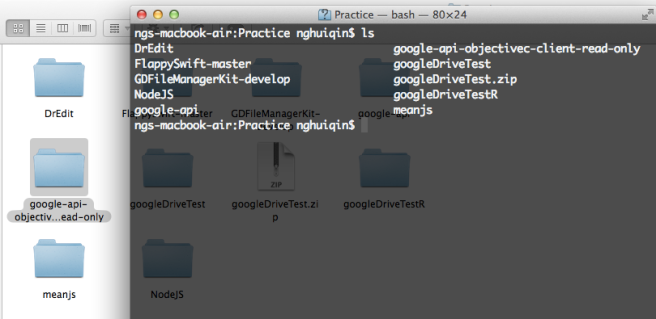
Go to google-api-objectivec-client-read-only/Source Run the GTL.xcodeproj 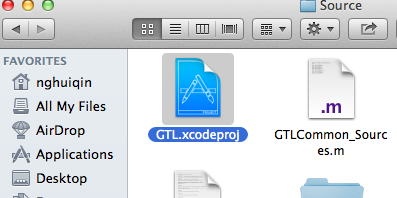
Build the project by running CMD+B

Then open your own project, here is an advice to create a folder(group) in the project 
Remember to link the path

Go back to your GTL project, expand the folders under GTL Source/Common except a folder Mac
Here is the magic
Select from Common folder to the bottom, and drag to your project’s GTL folder.

Remember to tick copy items
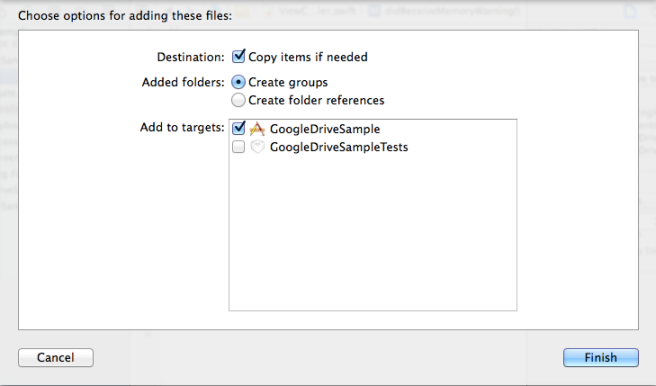
Select your project, go to Build Phases -> Compile Sources and select all the files with prefix GTL and GTM
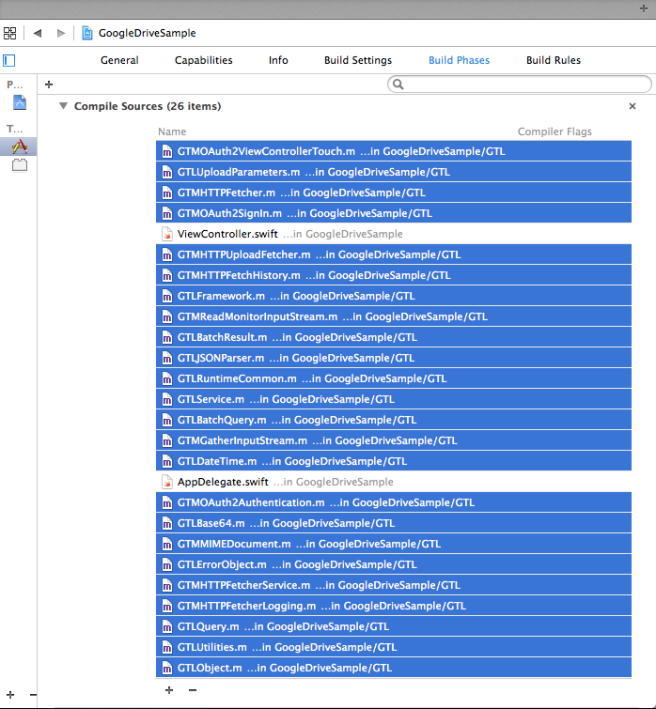
Press enter and there will have a dialog box prompt out, key in -fno-objc-arc and press enter again to add compiler flags.

Use same method to create another group for Google Drive
Go back to your google-api-objectivec-client-read-only downloaded from SVN
Under google-api-objectivec-client-read-only/Source/Services/Drive/Generated
select all files except GTLDrive_Sources.m drag and copy to your project

Well almost done! All You have to do now is adding the necessary framework into your project.
Security.framework
SystemConfiguration.framework

Ta-Da, the google drive library is set. You can build the project now if it doesn’t prompt any issue, then the library is successfully integrated!!
The rest, let’s run the example code to see whether it’s work!
For Swift project, remember to build your bridge header file, and import following files
#import "GTMOAuth2ViewControllerTouch.h"
#import "GTLDrive.h"
In order not to make my post longer, I have put the sample code in github, please download from here.